Black Firday Offer
Buy now with a special offer
What you will learn?
Create a beautiful animated todo list application
Create your own Node modules
Make REAL web applications using cutting-edge technologies
Create a complicated yelp-like application from scratch
Understand the ins and outs of HTTP requests
About this course
Rocket LMS is an online course marketplace with a pile of features that helps you to run your online education business easily. This product helps instructors and students to get in touch together and share knowledge.
Instructors will be able to create unlimited video courses, live classes, text courses, projects, quizzes, files, etc and students will be able to use the educational material and increase their skill level.
Rocket LMS is based on real business needs, cultural differences, advanced user researches so the product covers your business requirements efficiently.
Suggested by top companies
Top companies suggest this course to their employees and staff.
.png)
.png)
.png)
.png)
Requirements
Prepare to build real web apps!
Be ready to learn an insane amount of awesome stuff
Have a computer with Internet
FAQ
Comments (0)
iSpring Suite is a PowerPoint-based authoring toolkit produced by iSpring Solutions that allows users to create slide-based courses.
Adobe Captivate is an authoring tool that is used for creating eLearning content such as software demonstrations, software simulations, branched scenarios, and randomized quizzes in Shockwave Flash and HTML5 formats.
Always remember to tune "up" to a pitch and not down to a pitch. This helps in keeping your guitar in tune.
This course will teach you how to master your fretboard, understand music theory, and most importantly, how to use these tools to craft your unique sound.
YouTube is an American online video sharing and social media platform owned by Google. It was launched on February 14, 2005, by Steve Chen, Chad Hurley, and Jawed Karim.
Vimeo, Inc. is an American video hosting, sharing, and services platform provider headquartered in New York City. Vimeo focuses on the delivery of high-definition video across a range of devices. Vimeo's business model is through software as a service.
Rocket LMS mobile app is an Android mobile application for Rocket LMS. Rocket LMS is an online course marketplace that helps you run your online education business easily.
Google Drive is a file storage and synchronization service developed by Google. Launched on April 24, 2012, Google Drive allows users to store files in the cloud, synchronize files across devices, and share files.
Microsoft PowerPoint is a presentation program, created by Robert Gaskins and Dennis Austin at a software company named Forethought, Inc. It was released on April 20, 1987, initially for Macintosh computers only.
Portable Document Format (PDF), standardized as ISO 32000, is a file format developed by Adobe in 1992 to present documents, including text formatting and images, in a manner independent of application software, hardware, and operating systems.
Rocket LMS mobile app is an Android mobile application for Rocket LMS. Rocket LMS is an online course marketplace that helps you run your online education business easily.
March 20 is the first day of spring for most people, while March 1 is the first spring day for meteorologists.
Amazon S3 or Amazon Simple Storage Service is a service offered by Amazon Web Services that provides object storage through a web service interface. Amazon S3 uses the same scalable storage infrastructure that Amazon.com uses to run its global e-commerce network.
Amazon S3 or Amazon Simple Storage Service is a service offered by Amazon Web Services that provides object storage through a web service interface. Amazon S3 uses the same scalable storage infrastructure that Amazon.com uses to run its global e-commerce network.
A Homework to test yourself on your grasp of CSS and reassure yourself that you've got this!
Please send your homework as soon as possible.
Regards.
Quiz & Certificates

Reviews (1)
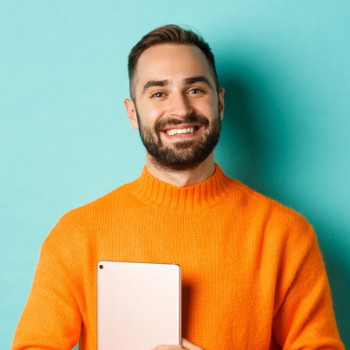